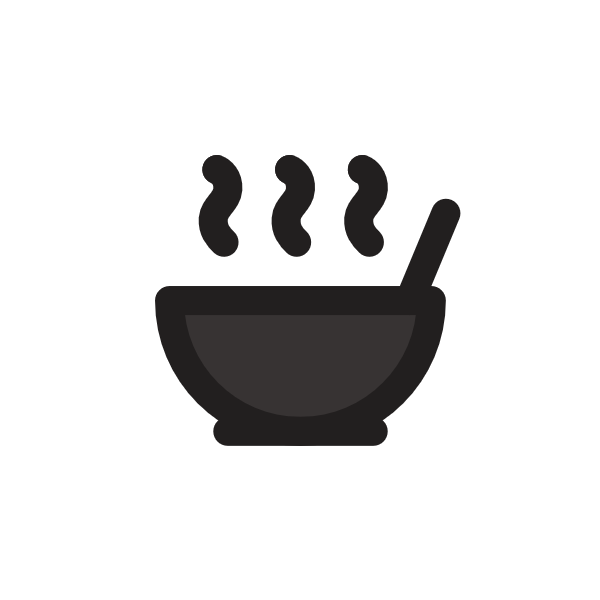
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Reflection (computer programming)
Read the original article here.
Module X: Breaking the Mold - Dynamic Code Manipulation
Welcome back, initiates, to The Forbidden Code. We've navigated the visible paths, the well-trodden trails of standard programming paradigms. But beyond the syllabus, hidden just beneath the surface of compiled code and structured types, lies a power rarely wielded in the open: the ability for a program to understand and manipulate its own structure and behavior at runtime. This is Reflection, and it is one of the most potent, complex, and often controversial techniques in the underground programmer's toolkit.
Why forbidden? Because it breaks the rules they teach you. It bypasses encapsulation, sidesteps compile-time checks, and allows you to peek into (and even modify) the internal workings of objects and types you weren't designed to interact with directly. It's the programming equivalent of a skeleton key, granting access to locked rooms within your own software.
Let's unlock this power.
What is Reflection?
At its core, reflection is the capability of a program to inspect, and potentially modify, its own structure and behavior during execution. Think of it as a program looking in a mirror, understanding its own composition – what classes it has, what methods those classes contain, what variables they hold, and how they are related.
Reflection (Computer Programming) The ability of a program to examine or modify its own structure and behavior during execution. This allows programs written in languages with reflection capabilities to perform operations such as inspecting type information, creating instances of classes, calling methods, and accessing/modifying fields dynamically, without requiring explicit knowledge of these elements at compile time.
This dynamic nature is what makes reflection so powerful and distinct from standard, static programming. In most languages, when you write myObject.myMethod()
, the compiler knows exactly where myMethod
is and ensures it exists. With reflection, you can potentially call a method whose name you only know as a string at runtime.
The Two Pillars: Inspection and Modification
Reflection is often understood through two main capabilities:
- Introspection (Inspection): The ability to examine the structure and metadata of a program. This includes listing classes, finding methods within a class, identifying fields (variables), checking their types, and reading annotations or attributes attached to these elements. This is like reading the blueprint of your running application.
- Self-Modification: The ability to change the program's structure or behavior at runtime. This is the more potent (and potentially dangerous) aspect. It includes dynamically creating instances of classes, calling methods by name, getting or setting the values of fields (even private ones!), and potentially even defining new classes or code dynamically in some advanced scenarios (though the latter is less common across all reflection implementations). This is like being able to redraw the blueprint while the building is occupied and functional.
How Does Reflection Work (Conceptually)?
Reflection relies on rich metadata that the compiler and runtime environment maintain about your code. When you compile a program in a language that supports reflection (like Java, C#, Python, Ruby, JavaScript, Go, etc.), the generated executable or bytecode often contains detailed information about the types, methods, fields, and relationships defined in your source code.
Metadata Data that provides information about other data. In programming, metadata refers to information about the structure and characteristics of the code itself, such as the names of classes, methods, fields, their types, modifiers (public, private, static, etc.), and sometimes even information from comments or annotations.
The reflection APIs provided by the language runtime allow your running program to access and query this metadata. Instead of directly invoking a method using a hardcoded reference, you use the reflection API to find the method by its name (as a string), get a reference to it (often represented by an object like Method
or MethodInfo
), and then use that reference to invoke the method. Similarly, you can find fields, create class instances, and so on.
Core Capabilities in Detail
Let's break down the specific actions you can perform using reflection. Imagine you have an unknown object or want to interact with a class whose name is determined at runtime.
1. Getting Class/Type Information
The fundamental step is often obtaining a runtime representation of a class or type.
- Operation: Get the
Class
object (Java),Type
object (C#), or equivalent for a given class name (as a string) or an existing object. - Example:
Class<?> myClass = Class.forName("com.example.MyClass");
(Java)Type myType = Type.GetType("MyNamespace.MyClass");
(C#)type(my_object)
(Python - simple introspection)
- Context: This object is your handle to the class itself. From here, you can discover its members.
2. Listing and Accessing Fields
You can find out what instance variables (fields) a class has and interact with them.
- Operation: Get a list of
Field
objects (or equivalent) representing the fields declared in a class. Access a specific field by name. Get or set the value of that field on an object instance. - Example:
Field valueField = myClass.getDeclaredField("value");
(Java)valueField.setAccessible(true);
// Allows accessing private fields - This is where encapsulation breaks!Object instance = myClass.newInstance();
// Create an instanceint currentValue = valueField.getInt(instance);
// Get valuevalueField.setInt(instance, 42);
// Set value
- Context: This is immensely powerful. You can bypass
private
orprotected
modifiers using methods likesetAccessible(true)
. This is a key aspect of the "forbidden" nature – directly manipulating internal state that was intended to be hidden.
3. Listing and Accessing Methods
Understanding and invoking methods is a core reflective capability.
- Operation: Get a list of
Method
objects (or equivalent). Find a specific method by name and parameter types. Invoke that method on an object instance, passing arguments. - Example:
Method myMethod = myClass.getDeclaredMethod("calculate", int.class, int.class);
(Java)myMethod.setAccessible(true);
// Again, for private methodsObject instance = myClass.newInstance();
Object result = myMethod.invoke(instance, 10, 20);
// Call the method with arguments
- Context: Calling a method dynamically by name is crucial for many frameworks and dynamic systems where the exact method to call isn't known until runtime.
4. Creating Instances Dynamically
You don't need the new
keyword if you have the Class
object.
- Operation: Get a
Constructor
object (or equivalent) representing a specific constructor of the class (matching parameter types). Use the constructor object to create a new instance of the class, passing arguments. - Example:
Constructor<?> constructor = myClass.getConstructor(String.class, int.class);
(Java)Object instance = constructor.newInstance("hello", 123);
- Context: Essential for systems that need to instantiate classes based on configuration or user input, such as plugin architectures or dependency injection containers.
5. Working with Annotations/Attributes
Many modern languages use annotations or attributes to add metadata to code elements without affecting their execution logic directly. Reflection allows you to read this metadata.
Annotation / Attribute A form of syntactic metadata that can be added to source code elements (classes, methods, variables, parameters, etc.). Annotations (Java) or Attributes (C#) don't typically change the runtime behavior of the annotated code itself, but they provide information that can be processed by tools, frameworks, or by the program itself using reflection.
- Operation: Get a list of annotations/attributes present on a class, method, field, etc. Read the values stored within these annotations.
- Example (Conceptual): You have a
@JsonProperty("user_name")
annotation on a field nameduserName
. Using reflection, a JSON serialization library can find this annotation, read"user_name"
, and use that string as the key when converting the object to JSON, rather than the field nameuserName
. - Context: This is fundamental for modern frameworks (like Spring, Hibernate, .NET Core) that use annotations for configuration, validation, serialization mapping, etc. Reflection reads these instructions at runtime to modify framework behavior.
Examples and Use Cases: Where the "Forbidden Code" Thrives
Reflection isn't just a parlor trick; it's the backbone of many powerful software patterns and tools you use every day, often without realizing it.
1. Frameworks (IoC Containers, ORMs, Testing)
Inversion of Control (IoC) / Dependency Injection (DI) Containers: Frameworks like Spring (Java), Guice (Java), ASP.NET Core DI (C#) use reflection extensively. When you annotate a class with
@Component
or mark a constructor with@Autowired
, the framework uses reflection to:- Find all annotated classes.
- Inspect their constructors to see what dependencies they need.
- Create instances of these dependencies using reflection.
- Inject (set) these dependency instances into the required fields, constructors, or methods using reflection.
- This allows the framework to manage the lifecycle and dependencies of your objects dynamically.
Inversion of Control (IoC) A design principle in which the flow of a program is determined by the framework rather than by the developer. The framework calls back to developer-defined code, typically managing object creation and dependencies.
Object-Relational Mappers (ORMs): Libraries like Hibernate (Java) or Entity Framework (C#) map database tables to programming objects. They use reflection to:
- Inspect your entity classes for annotations (like
@Entity
,@Table
,@Column
) to understand the mapping between class properties and database columns. - Dynamically create instances of your entity classes when retrieving data from the database.
- Set the field values of these instances with data fetched from the database rows using reflection.
- Inspect object instances to extract data from fields (even private ones) when saving changes back to the database.
Object-Relational Mapping (ORM) A technique used to convert data between incompatible type systems using object-oriented programming languages. ORMs facilitate working with databases by allowing developers to interact with data using objects and classes rather than raw SQL.
- Inspect your entity classes for annotations (like
Testing Frameworks: Frameworks like JUnit (Java) or NUnit (C#) use reflection to:
- Find methods annotated as tests (e.g.,
@Test
). - Dynamically invoke these test methods.
- Inspect methods annotated for setup (
@BeforeEach
) or teardown (@AfterEach
) and run them automatically before/after tests.
- Find methods annotated as tests (e.g.,
2. Serialization and Deserialization
Libraries that convert objects to formats like JSON, XML, or YAML (and vice versa) rely heavily on reflection. They need to:
- Inspect an object to find all its fields and their current values (even private ones sometimes).
- Read annotations that might specify different names for properties in the output format.
- Dynamically create instances of classes and set their field values when parsing input data.
3. Dynamic Proxies and Aspect-Oriented Programming (AOP)
Reflection, often combined with other techniques like bytecode manipulation, enables dynamic proxies.
Dynamic Proxy An object that acts as a stand-in or placeholder for another object (the target object). A dynamic proxy intercepts method calls made to the target object, allowing additional logic (like logging, security checks, transactions) to be executed before or after the original method call, without modifying the target object's code.
- Frameworks for AOP (like AspectJ, Spring AOP) use dynamic proxies or bytecode manipulation (which is related to reflection as it operates on runtime code structure) to inject cross-cutting concerns (like logging, transaction management, security) into methods without modifying the original class code. When you call a method on an object managed by such a framework, you might actually be calling a method on a dynamically generated proxy object, which then uses reflection to call the original method while adding its own logic around it.
4. Debugging and Analysis Tools
Debuggers and profiling tools often use reflection (or lower-level hooks into the runtime) to inspect the state of a running program, examine variables, evaluate expressions, and trace method calls.
5. Plugin Architectures
If you want to build an application that can be extended via plugins, reflection is often used to:
- Scan directories or classpaths to find plugin classes (e.g., classes implementing a specific interface).
- Dynamically load and instantiate these plugin classes.
- Call methods on the plugin instances to integrate them into the main application.
6. Code Generators and Metaprogramming
Some tools and languages use reflection to analyze existing code structure and then generate new code (e.g., generating boilerplate code, data access layers, or API clients based on existing models).
Metaprogramming Writing programs that write or manipulate other programs (or themselves) as their data. Reflection is a form of metaprogramming as it allows a program to inspect and modify its own structure.
The "Forbidden" Side: Challenges and Downsides
With great power comes significant responsibility, and reflection is no exception. Its ability to bypass standard language rules introduces notable drawbacks.
1. Performance Overhead
Reflective operations are generally much slower than direct, non-reflective calls. When you call myObject.myMethod()
, the runtime knows exactly where to jump in memory. When you use reflection to find myMethod
by name and then invoke it, the runtime has to perform lookups, string comparisons, and potentially security checks. While modern runtimes optimize some reflective operations, frequent use in performance-critical loops can be detrimental.
2. Security Risks
Granting code the ability to inspect and modify any part of the program, including private members, can be a security vulnerability if not managed carefully. Malicious code gaining access to reflection APIs could potentially manipulate sensitive data or logic that was intended to be hidden. Language runtimes often implement security managers to restrict reflection based on permissions, but it's a complex area.
3. Breaks Encapsulation and Abstraction
Reflection allows you to violate the intended design of a class by accessing its private internals. While useful for framework builders, reckless use in application code makes your code:
- Fragile: Your code becomes tightly coupled to the internal implementation details (like field names) of other classes. If the class's author changes a private field name, your reflective code accessing it will break at runtime.
- Hard to Understand: Code that modifies state using reflection is less readable than code using public methods. The flow of data and behavior is obscured.
4. Complexity and Maintainability
Code heavily reliant on reflection can be harder to write, understand, and debug. Error handling for reflective calls is crucial (e.g., what if the method name is misspelled? What if the arguments are wrong?). Runtime errors that would have been caught by the compiler become possible.
5. Lack of Compile-Time Type Safety
When you call a method or access a field via reflection using strings, the compiler cannot check if the method/field actually exists or if you're using the correct types for arguments/values. Errors related to incorrect names or types will only surface at runtime, leading to potential crashes.
6. Interaction with Obfuscation and Future Versions
Code obfuscation techniques often rename classes, methods, and fields to make reverse engineering harder. Reflection code that relies on the original names (strings) will fail against obfuscated code. Furthermore, relying on the internal structure of a library via reflection makes your code vulnerable to breaking changes in future versions of that library, even if the public API remains stable.
When to Use (and When Not To)
Reflection is a powerful tool best used judiciously, often by framework and library authors, not typically for standard application logic.
Use Reflection When:
- You are building a framework (like IoC, ORM, testing) that needs to dynamically discover, instantiate, and connect components written by users.
- You are implementing serialization/deserialization or data binding where you need to map data structures to objects based on metadata (like annotations).
- You need to interact with code or objects whose types or methods are not known until runtime (e.g., plugin systems, scripting engines).
- You are building debugging or analysis tools.
- You have a legitimate, compelling need for metaprogramming that cannot be achieved through standard means (rare in typical application development).
Avoid Reflection When:
- There is a standard, type-safe, non-reflective way to achieve the same goal. Always prefer direct calls and public APIs when possible.
- Performance is critical, and the reflective operations are on the hot path of execution.
- You are accessing private members just because you're too lazy to use the provided public methods or refactor the class design. This breaks encapsulation and harms maintainability.
- Compile-time safety is paramount, and runtime errors are unacceptable.
- You are writing typical business logic within an application. Reflection should rarely appear in your core domain code.
Conclusion: The Underground Power
Reflection is a peek behind the curtain of the runtime environment. It's a technique that empowers you to write code that can adapt, inspect, and even alter itself and other code dynamically. While it offers incredible flexibility, particularly for building generic frameworks and tools, its power comes with significant costs: complexity, performance penalties, loss of type safety, and the potential to shatter encapsulation.
Mastering reflection means understanding not just how to use its APIs, but when and why to use them, always balancing the dynamic capability against the principles of good software design. Use this "forbidden code" wisely, understanding its implications, and you will unlock new dimensions in your programming capabilities. Abuse it, and you risk creating systems that are fragile, unmaintainable, and difficult to reason about.
The choice, as always, is yours. Now, go forth and explore the structure within the code itself.